10.1 📖 概述
💬 说明
直接内存不是虚拟机运行时数据区的一部分,也不是《Java虚拟机规范》中定义的内存区域。
直接内存是在Java堆外的,直接向系统申请的内存区间。
来源于NIO,通过存在堆中的DirectByteBuffer操作Native内存。
⚙️ 设置
- 直接内存大小可以通过
MaxDirectMemorySize
设置
- 如果不指定,默认与堆的最大值
-Xmx
参数值一致
🌠 特点
通常,访问直接内存的速度会优于Java堆,即读写性能高。
- 因此出于性能考虑,读写频繁的场合可能会考虑使用直接内存。
- Java的NIO库允许Java程序使用直接内存,用于数据缓冲区。
⌨️ 示例
使用下列代码,直接分配本地内存空间
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| public class BufferTest {
private static final int BUFFER = 1024 * 1024 * 1024;
public static void main(String[] args) { ByteBuffer byteBuffer = ByteBuffer.allocate(BUFFER); System.out.println("直接内存分配完毕"); Scanner scanner = new Scanner(System.in); scanner.next(); System.out.println("直接内存开始释放"); byteBuffer = null; System.gc(); } }
|
运行之后可以看到进程已分配内存:
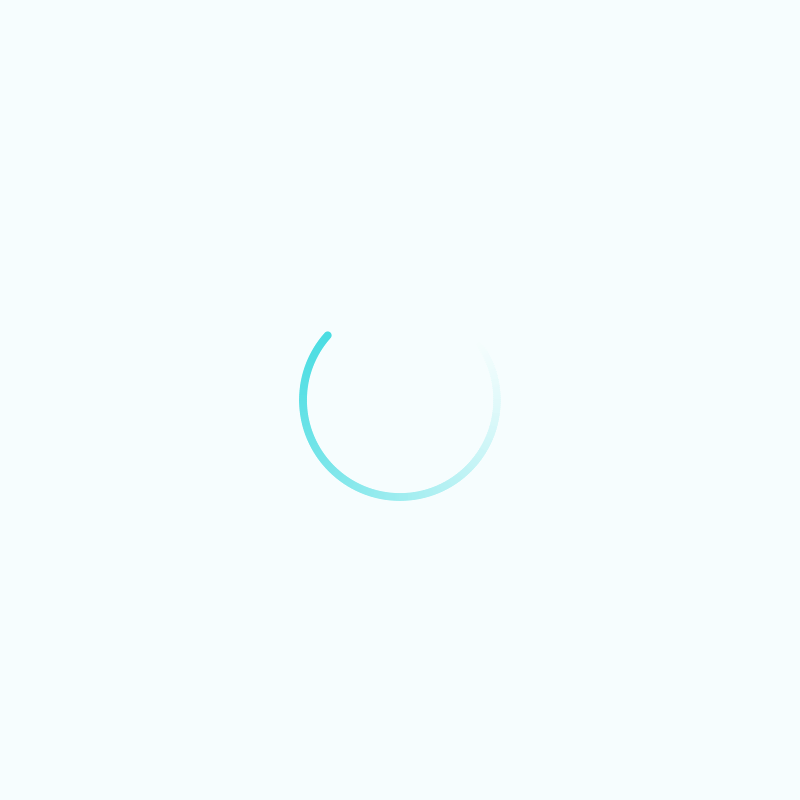
10.2 🌀 非直接缓冲区和缓冲区
🟣 非直接缓冲区
读写文件,需要与磁盘交互,需要由用户态切换到内核。在内核态时,需要内存如下图的操作。
需要两份内存存储重复数据,效率低。
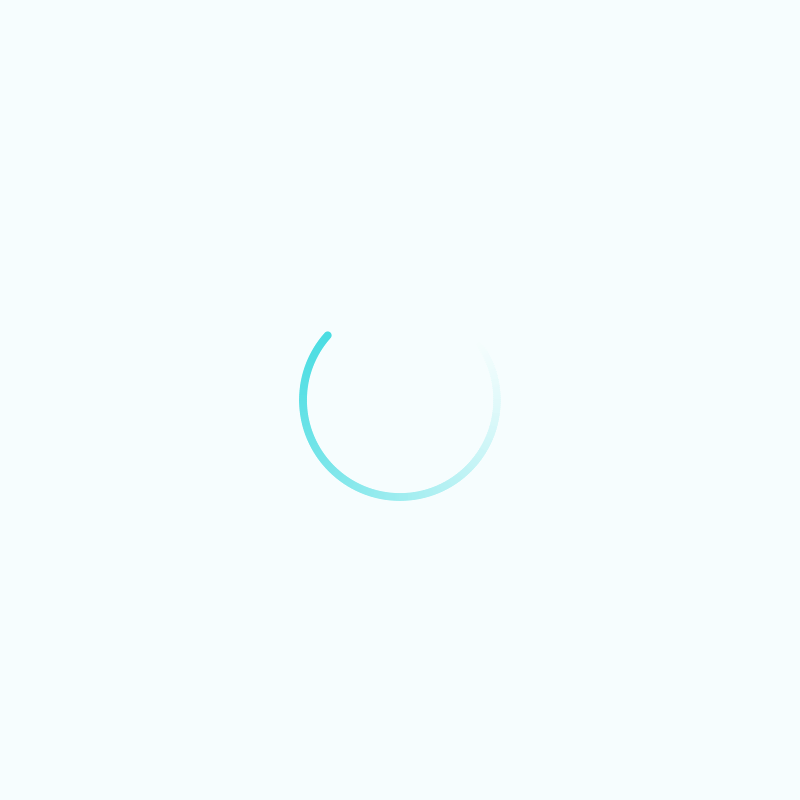
🟠 直接缓冲区
使用NIO时,如下图。
操作系统划出的直接缓存区可以被Java代码直接访问,只有一份。
NIO适合对大文件的读写操作。
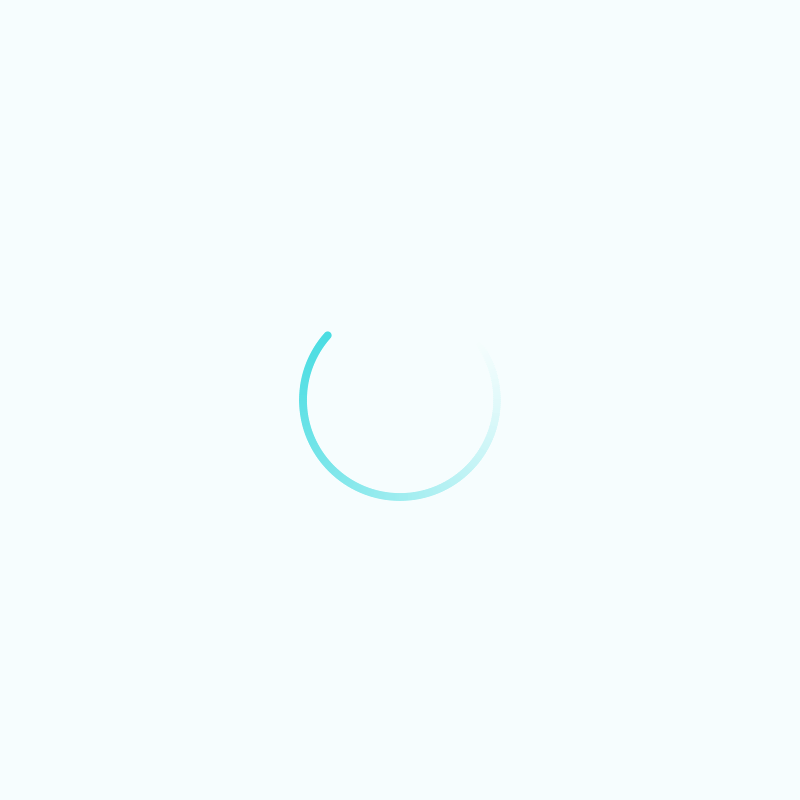
⌨️ 测试案例
分别使用BIO和NIO进行复制复联四电影文件(3.74GB):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| public class CopyFileTest {
private static final String MOVIE_NAME = "D:/BaiduNetdiskDownload/复仇者联盟4-终局之战.mp4"; private static final int ONE_HUNDRED_MB = 1024 * 1024 * 100;
public static void main(String[] args) throws IOException { System.out.println("BIO耗时:" + inDirectBuffer(MOVIE_NAME, MOVIE_NAME + ".copy.mp4")); System.out.println("NIO耗时:" + directBuffer(MOVIE_NAME, MOVIE_NAME+ ".copy.mp4")); }
private static long inDirectBuffer(String src, String dest) throws IOException{ long start = System.currentTimeMillis(); FileInputStream inputStream = new FileInputStream(src); FileOutputStream outputStream = new FileOutputStream(dest); byte[] buffer = new byte[ONE_HUNDRED_MB]; int len = 0; while ((len = inputStream.read(buffer)) != -1) { outputStream.write(buffer, 0, len); } long end = System.currentTimeMillis(); inputStream.close(); outputStream.close(); return end - start; }
private static long directBuffer(String src, String dest) throws IOException{ long start = System.currentTimeMillis(); FileChannel inChannel = new FileInputStream(src).getChannel(); FileChannel outChannel = new FileOutputStream(dest).getChannel(); ByteBuffer byteBuffer = ByteBuffer.allocate(ONE_HUNDRED_MB); while (inChannel.read(byteBuffer) != -1) { byteBuffer.flip(); outChannel.write(byteBuffer); byteBuffer.clear(); } long end = System.currentTimeMillis(); inChannel.close(); outChannel.close(); return end - start; } }
|
运行结果:
10.3 💢 存在的问题
🔺 问题
🔻 缺点
💌 总结
java process memory = java heap + native memory
进程内存空间 = 堆空间 + 本地内存